How To Integrate Mopub Native Ads Swift
Native ads let you monetize your app via a UI ad component that is consistent with your app’s design.
Prerequisites
Before integrating native ads into your app, create an ad account on Mopub’s website and download the SDK using Cocoapod.
pod 'mopub-ios-sdk' # Mopub SDK
For Ad adapters such as Facebook, Admob and Pangle mediation add:
pod 'MoPub-FacebookAudienceNetwork-Adapters' # facebook Mopub adapter
pod 'MoPub-AdMob-Adapters' # Admob Mopub adapters
pod 'MoPub-Pangle-Adapters' # Pangle Mopub adapters
For a full of available adapters, please visit here.
Run pod install in your terminal.
Testing Ads
It’s always advised you use a test ad unit when testing your apps. Testing your app using live ads could lead to your account being terminated or suspended. Mopub‘s available test ads:
It’s always advised you use a test ad unit when testing your apps. Testing your app using live ads could lead to your account being terminated or suspended. Mopub’s available test ads:
Format | Size | Ad unit ID |
---|---|---|
Banner | 320×50 | 0ac59b0996d947309c33f59d6676399f |
Banner (MRect) | 300×250 | 2aae44d2ab91424d9850870af33e5af7 |
Interstitial | 320×480 | 4f117153f5c24fa6a3a92b818a5eb630 |
Rewarded Ad | 320×480 | 8f000bd5e00246de9c789eed39ff6096 |
Rewarded Playable (MRAID) | 320×480 | 98c29e015e7346bd9c380b1467b33850 |
Native | N/A | 76a3fefaced247959582d2d2df6f4757 |
Load Ad
The first step in integrating your ad is by initialising it in your App Delegate. To initialise your ads, import Mopub and configure your ad units.
import MoPubSDK // Mopub ad
// Uncomment this line for Adapters
// import MoPub_FacebookAudienceNetwork_Adapters // Facebook adapter
// import MoPub_Pangle_Adapters // Pangle mobile adapters
// import MoPub_AdMob_Adapters // Mopub adapters
@UIApplicationMain
class AppDelegate: UIResponder, UIApplicationDelegate {
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplicationLaunchOptionsKey: Any]?) -> Bool {
// Mopub ad network
let sdkConfig = MPMoPubConfiguration(adUnitIdForAppInitialization: "YOUR_AD_UNIT")
sdkConfig.loggingLevel = .none
sdkConfig.allowLegitimateInterest = true
/* Uncomment this line for Adapters
let pangleConfig: NSMutableDictionary = [
"app_id": "YOUR_APP_ID"
]
let facebookConfig: NSMutableDictionary = [
"native_banner": "true"
]
let googleConfig: NSMutableDictionary = [
"native_banner": "true"
]
sdkConfig.additionalNetworks = [PangleAdapterConfiguration.self]
sdkConfig.additionalNetworks = [AppLovinAdapterConfiguration.self]
sdkConfig.additionalNetworks = [FacebookAdapterConfiguration.self]
sdkConfig.additionalNetworks = [GoogleAdMobAdapterConfiguration.self]
sdkConfig.mediatedNetworkConfigurations = [
"PangleAdapterConfiguration": pangleConfig,
"FacebookAdapterConfiguration": facebookConfig,
"GoogleAdMobAdapterConfiguration": googleConfig
]
*/
// Mopub Configuration
MoPub.sharedInstance().grantConsent()
MoPub.sharedInstance().allowLegitimateInterest = false
MoPub.sharedInstance().initializeSdk(with: sdkConfig, completion: nil)
}
}
Using an ad placer is generally the best way to integrate native ads. This option automatically wraps the TableView and CollectionView data source and delegate.
In this documentation, we’ll largely be focusing on using the ad placer on a UICollectionView but the principle is similar to UITableView (i.e. change “CollectionView” to “TableView”). If you would like to integrate native ads manually please visit Mopub’s documentation.
Prepare the variables and class.
import MoPubSDK // Mopub
class ViewController: UIViewController, UICollectionViewDelegate, UICollectionViewDataSource, UICollectionViewDelegateFlowLayout UITableViewDelegate, MPCollectionViewAdPlacerDelegate {
// For mobile ads
let collectionViewVH: UICollectionView? // Your Collection either created manually or via storyboard
var adNativeUnit = "YOUR_AD_UNIT"
var nativeAd: MPNativeAdRequest?
var placer: MPTableViewAdPlacer?
var adPosition = MPServerAdPositioning()
}
In your view did load, set your CollectionView delegate and data source to Mopubs delegate and data source as seen below. The final step in prepare your data source is refreshing using Mopub’s reload function.
override func viewDidLoad() {
super.viewDidLoad()
// Set mopub ad delegate and data source
self.collectionViewVH.mp_setDelegate(self)
self.collectionViewVH.mp_setDataSource(self)
// Function to prepare ads
self.loadNativeAd()
// Usually called when your content has been loaded e.g via a server request
// For this demo it is placed here
self.collectionViewVH.mp_reloadData()
}
For ease of use and recyclability, the steps in loading native ads have been kept in function (loadNativeAd) and is called in viewDidLoad().
func loadNativeAd() {
// Your ads assets and targetting
let targeting: MPNativeAdRequestTargeting! = MPNativeAdRequestTargeting()
targeting.desiredAssets = Set([kAdIconImageKey,kAdMainImageKey, kAdCTATextKey, kAdTextKey, kAdTitleKey])
let positioning:MPAdPositioning = MPAdPositioning()
let settings:MPStaticNativeAdRendererSettings = MPStaticNativeAdRendererSettings()
// Set the rendering class to your collection view cell class.
settings.renderingViewClass = YOUR_CELL_CLASS.self
settings.viewSizeHandler = {(maxWidth: CGFloat) -> CGSize in
let paddingSpace =
let availableWidth = YOUR_CELL_HEIGHT
let widthPerItem = YOUR_CELL_WIDTH
let size:CGSize = CGSize(width: widthPerItem, height: widthPerItem)
return size
}
// Intervals at which you want your ad to show e.g at every 6th item.
positioning.repeatingInterval = 6
// Adapters configuration
let mpConfig:MPNativeAdRendererConfiguration = MPStaticNativeAdRenderer.rendererConfiguration(with: settings)
mpConfig.supportedCustomEvents = ["MPMoPubNativeCustomEvent"]
/* Uncomment this
let fbConfig:MPNativeAdRendererConfiguration = FacebookNativeAdRenderer.rendererConfiguration(with: settings)
fbConfig.supportedCustomEvents = ["MPMoPubNativeCustomEvent"]
let pngleConfig: MPNativeAdRendererConfiguration = PangleNativeAdRenderer.rendererConfiguration(with: settings)
pngleConfig.supportedCustomEvents = ["MPMoPubNativeCustomEvent"]
let admobConfig: MPNativeAdRendererConfiguration = MPGoogleAdMobNativeRenderer.rendererConfiguration(with: settings)
admobConfig.supportedCustomEvents = ["MPMoPubNativeCustomEvent"]
self.placer = MPCollectionViewAdPlacer(collectionView: self.eventlistCollectionView, viewController: self, adPositioning: positioning, rendererConfigurations: [mpConfig, fbConfig, pngleConfig, admobConfig])
*/
// Remove this if you are using adapters and replace it with the above
self.placer = MPCollectionViewAdPlacer(collectionView: self.collectionViewVH, viewController: self, adPositioning: positioning, rendererConfigurations: [mpConfig])
self.placer?.delegate = self
self.placer?.loadAds(forAdUnitID: self.adNativeUnit, targeting: targeting)
}
func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let cell = collectionView.mp_dequeueReusableCell(withReuseIdentifier: "Your_Cell_ID", for: indexPath) as! YOUR_CELL_CLASS
}
That’s it, run your app in Xcode.
Going Live
Once you are ready to go live, change your app unit to the one you’ve created on Mopub.
To monitor your app’s monetisation, set targets and much more download our Motics app.
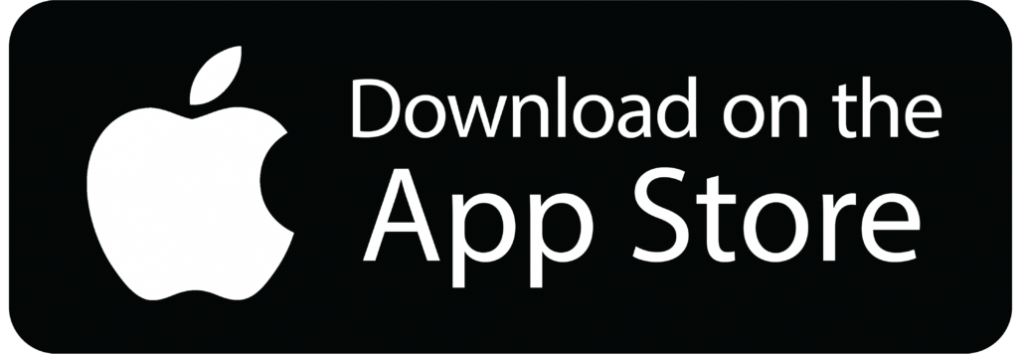